Setting up Mailchimp with your Next.js or React app
This simple guide helps you get your email marketing list with Mailchimp up and running quickly using Next.js or React.
•
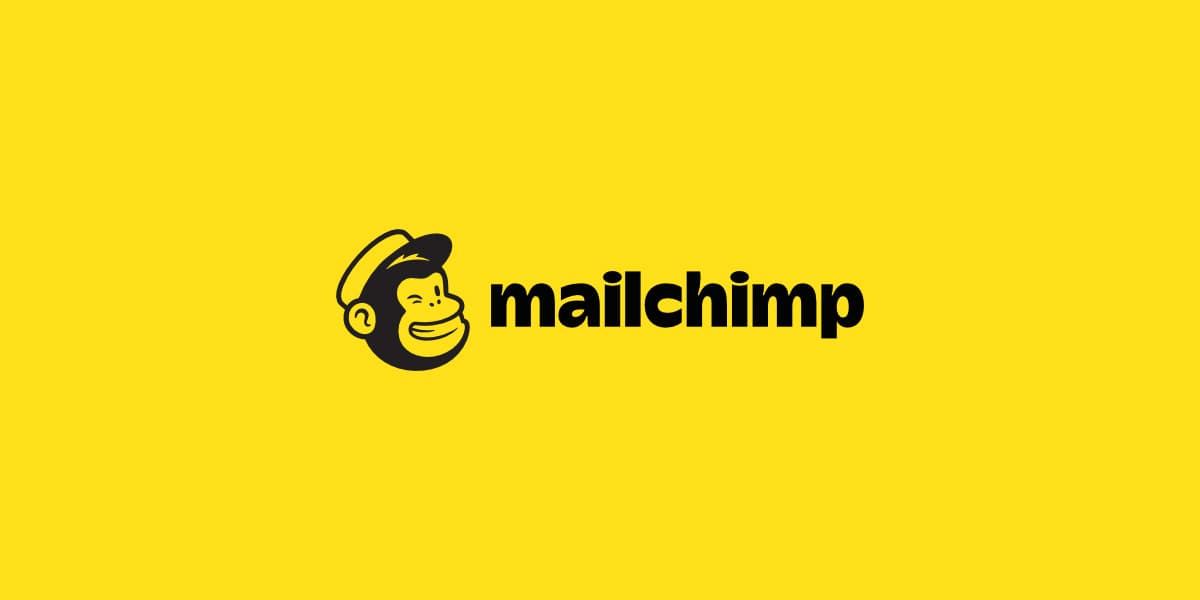
Alright, today we're going to write a little bit of code! I'm sharing the component that I'm going to use in the SaaS starter template for allowing your users to subscribe to a Mailchimmp email list.
We'll keep this short and sweet, here's what you need to do if you're running a TypeScript Next.js or React project:
Install dependencies
All we need to make sure this works correctly is the jsonp
package to make sure we can call Mailchimps API.
npm i --save jsonp
npm i --save-dev @types/jsonp
The component below also uses Tailwind, but you can take out my classes and customize it however you'd like.
Sign up for Mailchimp
Sign up over at Mailchimp. After you go through the signup process, you want to create an "Embedded Form".
Once you've created the form, Mailchimp will give you code to use it, but we're only going to take the <form>
action URL.
Copy yours and save it for later, it should look something like this:
"https://dev.us10.list-manage.com/subscribe/post?u=<ID>&id=<ID>&f_id=<ID>"
Add the Triple Threat Mailchimp component
Add the following component in src/components/tt-mailchimp-form.tsx
. Then replace <YOUR URL HERE>
with your
Mailchimp URL from the previous step.
import { useState } from "react";
import jsonp from "jsonp";
const yourMailchimpURL = '<YOUR URL HERE>';
function classes(...classes: string[]) {
return classes.filter(Boolean).join(' ')
}
interface TripleThreatMailchimpTypes {}
const TTMailchimpForm: React.FC<TripleThreatMailchimpTypes> = ({
}) => {
const [email, setEmail] = useState('');
const [submitting, setSubmitting] = useState(false);
const [status, setStatus] = useState('');
const [error, setError] = useState('');
const onSubmit = (e: any) => {
e.preventDefault();
e.stopPropagation();
setSubmitting(true);
setStatus('');
setError('');
let url = yourMailchimpURL;
url = url.replace('/post?', '/post-json?');
jsonp(`${url}&EMAIL=${email}`, { param: 'c' }, (err: any, data: any) => {
if (err) {
setStatus('error');
setError(err)
} else if (data.result !== "success") {
setStatus('error');
setError(data.msg);
} else {
setStatus('success');
setError(data.msg);
}
setSubmitting(false);
});
};
return (
<div>
<form onSubmit={onSubmit}
className={classes(status == 'success' ? "hidden" : "", "flex gap-3 pt-6 justify-center")}>
<input
type="email"
name="EMAIL"
placeholder="Your email"
className="px-3 rounded-full"
required
onChange={(e) => setEmail(e.target.value)}
></input>
<button type="submit" disabled={submitting}
className={`rounded-full bg-orange-500 disabled:bg-orange-200 px-3.5 py-2.5 text-xl text-white
font-semibold pl-6 pr-6 shadow-sm hover:bg-orange-600 focus-visible:outline focus-visible:outline-2
focus-visible:outline-offset-2 focus-visible:outline-orange-600`}>
Subscribe
</button>
</form>
<div className={classes(status == 'success' ? "" : "hidden", "flex gap-3 pt-6 justify-center")}>
<p className="text-lg leading-8">Thanks for subscribing!</p>
</div>
<div className={classes(status == 'error' ? "" : "hidden", "flex gap-3 pt-6 justify-center")}>
<p className="text-lg leading-8">There was an error subscribing you to our mailing list: {error}</p>
</div>
</div>
)
}
export default TTMailchimpForm;
Import and use your component
Now you can import and use the component with <TTMailchimpForm />
in any one of your pages!
Want to see a live demo? Scroll down to the bottom of this page to sign up for my mailing list and receive more product, marketing, and engineering tips weekly ;)
Until next time,

Subscribe to the newsletter.
Become a triple threat.
TripleThreat.dev teaches you the most impactful and essential Product, Marketing, and Engineering skills.
Thanks for subscribing!
There was an error subscribing you to our mailing list: